Theming Websites using Spring MVC
Many websites today allow their users to theme or change the look and feel of their sites. Gmail for example, currently provides over 34 themes to skin the mail interface. Themes can make websites more interactive and put the user in the driver seat when it comes to experiencing the site.
Conceptually, a theme is a collection of static resources such as stylesheets and images. When a user picks a theme, the theme’s styles and images dynamically gets associated with the site. In this post, I will create a simple Spring MVC Web application and show how Spring MVC can be used to manage theme resources easily. If you have used Spring MVC quite a bit, you can safely skip to Step 5.
Step 1: The first step in the process is to create a Spring MVC web project. Following maven conventions, here is the directory structure of the project:
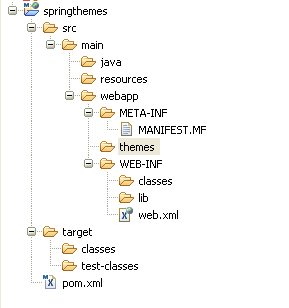
Spring MVC Theme Project Structure
Step 2: The next step is to add the required jars. Here is the dependency list that needs to be added to the pom.xml file:
<dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>2.5.6.SEC01</version> <scope>compile</scope> </dependency> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.14</version> <scope>compile</scope> </dependency> </dependencies>
This would add the following jars to the project:
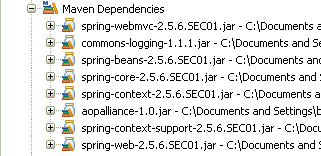
Spring MVC Theme Jars
Step 3: The next step is to add Spring “goodness” to the project. This is done by adding Spring MVC dispatcher servlet definition to web.xml:
<!-- Spring MVC Servlet --> <servlet> <servlet-name>springthemes</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springthemes</servlet-name> <url-pattern>*.html</url-pattern> </servlet-mapping>
The Spring MVC Dispatcher Servlet by default looks for the <context-name>-servlet.xml file for Web Tier bean definitions. Create a file called springthemes-servlet.xml under WEB-INF folder with the following information:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd"> <!-- Scan for controllers --> <context:component-scan base-package="com.inflinx.blog.springthemes.web.controller" /> <!-- Views are jsp pages defined directly in the root --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" p:suffix=".jsp"/> </beans>
Step 4: To keep things simple, create a controller class called HomeController that simply redirects to the home.jsp page. Here is the HomeController class definition:
package com.inflinx.blog.springthemes.web.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; @Controller @RequestMapping("/home.html") public class HomeController { @RequestMapping(method = RequestMethod.GET) public String showHome() { return "home"; } }
Here is the home.jsp file:
<html> <head> <title>Welcome to Spring Themes</title> </head> <body> Hello Visitor!! </body> </html>
If you deploy the application and hit http://<server:port>/springthemes/home.html, you should see a page that looks like this:
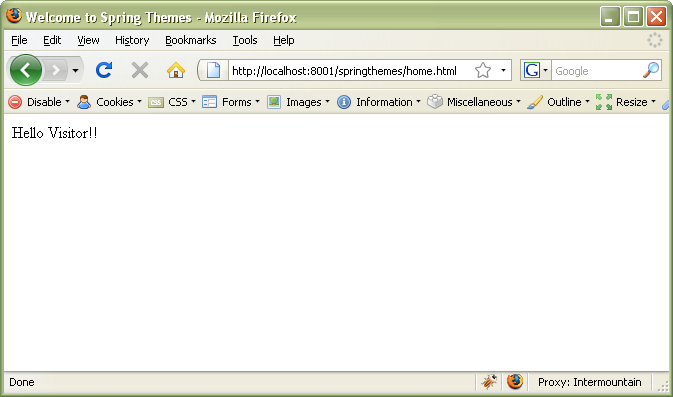
Spring MVC Theme Default Page
Now that we are successfull in creating a simple Spring MVC project, lets add two themes to it – dark theme and bright theme. The site automatically gets the “dark” theme during nights and the “bright” theme during day time.
Step 5: In this step, lets create the css files (one of the many static resources that a theme can have) associated with each theme. Here are the css files (I placed them under the themes folder):
dark.css
body { font-size:13px; text-align:center; color: white; background-color: black }
bright.css
body { font-size:9px; text-align:center; color: blue; background-color: white; }
Step 6: The next step is to define the themes. The default way to do this in Spring MVC is to use one property file for each theme. Here are the two theme definitions:
#dark theme properties file dark.properties
css=themes/dark.css
page.title=Welcome to Dark Theme
welcome.message=Hello Visitor!! Have a Good night!!
#bright theme properties file bright.properties
css=themes/bright.css
page.title=Welcome to Bright Theme
welcome.message=Hello Visitor!! Have a Good day!!
Step 7: Now that we have defined the themes, we need to tell Spring where to find them. This is done using a ThemeSource. Add the following line to the springthemes-servlet.xml file:
Step 8: Spring needs to know what theme to use when a request is made. This is done using a ThemeResolver. Spring provides three theme resolvers out of the box: FixedThemeResolver, SessionThemeResolver, CookieThemeResolver that are sufficient for most use cases. However, our site needs to inherit a theme based on the time, we will create a new theme resolver called DarkAndBrightThemeResolver:
package com.inflinx.blog.springthemes.web.resolver; import java.util.Random; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.web.servlet.theme.AbstractThemeResolver; public class DarkAndBrightThemeResolver extends AbstractThemeResolver { @Override public String resolveThemeName(HttpServletRequest arg0) { return isNight() ? "dark" : "bright"; } // Pretty lame implementation private boolean isNight() { return new Random().nextBoolean(); } @Override public void setThemeName(HttpServletRequest arg0, HttpServletResponse arg1, String arg2) { } }
Add the newly created theme resolver definition to the springthemes-servlet.xml file:
Step 9: The final step is use modify the view to use the theme. This is done using
<%@ taglib prefix="spring" uri="http://www.springframework.org/tags"%> <html> <head> <link rel="stylesheet" href='<spring:theme code="css"/>' type="text/css" /> <title><spring:theme code="page.title"/></title> </head> <body> <spring:theme code="welcome.message" /> </body> </html>
Now, redeploy the application and as you refresh the URL, you should randomly see the site switch between the two themes. Here is the site with the two themes:
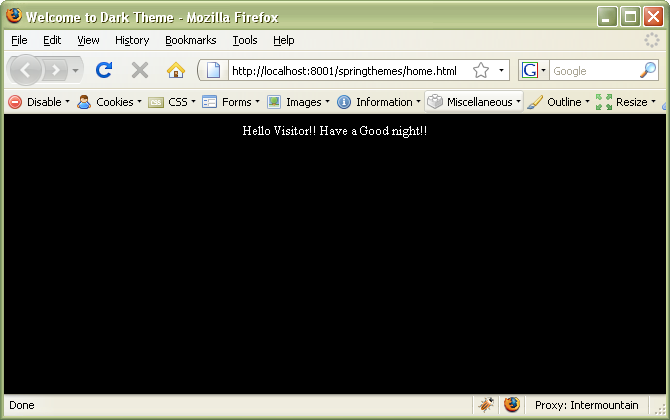
Dark Theme
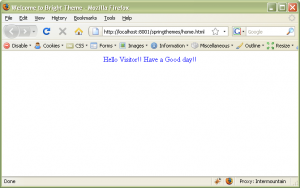
Bright Theme