EJB 3 Message Driven Beans in WebLogic 10.3
In this post, I will show how to create and test Message Driven Beans in WebLogic 10.3. Here are the steps:
Step 1: The first step is to create projects to hold Message Driven Beans. Using Eclipse IDE, here are the generated EAR and EJB projects:
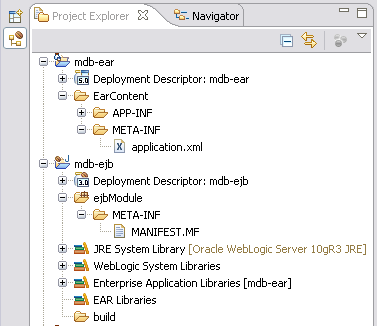
MDB Project Layout
EAR is the standard packaging mechanism for Java enterprise applications.
Step 2: The next step is to create the Message Driven Bean. The MDB created in this post will be consuming messages from a Distributed Queue with the JNDI name jndi.blogQueue. Refer to the “Distributed JMS Queue on WebLogic 10” post for details on creating Distributed Queues in WebLogic.
package com.inflinx.blog.springmdb; import javax.ejb.ActivationConfigProperty; import javax.ejb.MessageDriven; import javax.jms.Message; import javax.jms.MessageListener; @MessageDriven( mappedName = "jndi.blogQueue", activationConfig = { @ActivationConfigProperty( propertyName = "destinationType", propertyValue = "javax.jms.Queue" )} ) public class TestMdb implements MessageListener { public void onMessage(Message message) { System.out.println("Received Message: " + message); } }
Step 3: The next step is to package the newly created MDB into an EJB which then gets packaged into a ear file for deployment. During development in Eclipse, simply right click on the mdb-ear and click Run -> Run On Server. This IDE deployment assumes that the Oracle Server Adapter is installed in Eclipse and configured to talk to a WebLogic 10.3 server instance.
Step 4: To make sure that the MDB is deployed properly, let us create a MessageGenerator class that adds a message to the queue:
public class MessageGenerator { public static void main(String[] args) throws Exception { Context context = getInitialContext(); ConnectionFactory connectionFactory = (ConnectionFactory)context.lookup("jndi.blogfactory"); Queue queue = (Queue) context.lookup("jndi.blogQueue"); Connection connection = connectionFactory.createConnection(); Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE); MessageProducer producer = session.createProducer(queue); TextMessage message = session.createTextMessage(); message.setText("Hello World"); producer.send(message); connection.close(); } private static Context getInitialContext() throws Exception { Hashtable env = new Hashtable(); env.put(Context.INITIAL_CONTEXT_FACTORY, "weblogic.jndi.WLInitialContextFactory"); // TODO: Change the server and port name to suit your environment before running the class env.put(Context.PROVIDER_URL, "t3://localhost:9001"); return new InitialContext(env); } }
Running the above class will add a new TextMessage to the Queue. Once a new message is available, the TestMDB’s onMessage() method gets invoked and you should see the message “Received Message: TextMessage[ID:
For the above class to run from Eclipse, make sure that you remove “WebLogic System Libraries” and add weblogic.jar to the MessageGenerator “Run Configurations…”.
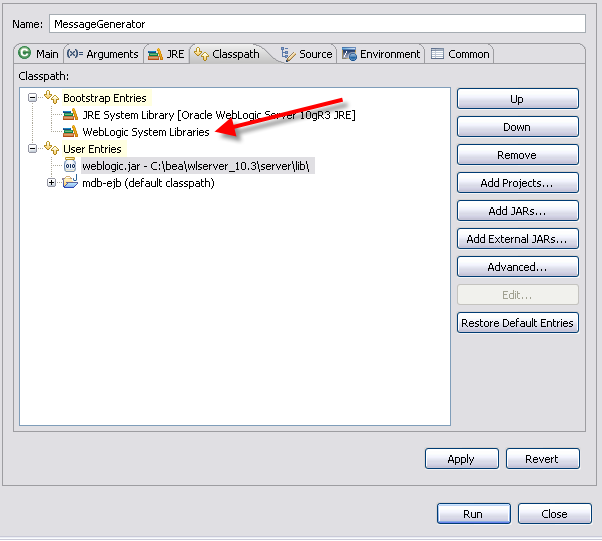
Message Generator Run Configuration
Otherwise you will end up getting the error:
Exception in thread “main” java.lang.NoClassDefFoundError: weblogic/kernel/KernelStatus
at weblogic.jndi.Environment.(Environment.java:78)
at weblogic.jndi.WLInitialContextFactory.getInitialContext(WLInitialContextFactory.java:117)
at javax.naming.spi.NamingManager.getInitialContext(NamingManager.java:667)
at javax.naming.InitialContext.getDefaultInitCtx(InitialContext.java:288)
at javax.naming.InitialContext.init(InitialContext.java:223)
at javax.naming.InitialContext.(InitialContext.java:197)
at com.inflinx.blog.test.MessageGenerator.getInitialContext(MessageGenerator.java:40)
at com.inflinx.blog.test.MessageGenerator.main(MessageGenerator.java:18)
Download the source code for this post here.